C Program To Implement Dictionary Using Hashing
- Dictionaries, Maps, and Hash Tables in Python.
- Hash Tables - Princeton University.
- Hash Table Program in C - Tutorialspoint.
- GitHub - HimanshuSingal/dictionary_hashing: C++.
- Dictionary using C - GitHub.
- Hashing function in C | Types of Collision Resolution.
- Implementing a phone directory in C++ - Coding Ninjas Blog.
- Implement Dictionary in C - Delft Stack.
- Algorithm and Examples of C++ Hash Table - EDUCBA.
- Hash Table in Data Structure: Python Example - Guru99.
- Problem statement implement all the functions of a.
- C Program To Implement Dictionary Using Hashing Algorithm.
- [Solved] C++ Hash Table In C++ , we will be implementing a.
Dictionaries, Maps, and Hash Tables in Python.
We will use an array of buckets to store the data, then use a hash function to turn a string into a number in the range 0...MAX_ELEMENTS. each bucket will hold a linked list of strings, so you can retrieve information again. Typically o (1) insertion and find.
Hash Tables - Princeton University.
Jan 31, 2013 · This program first reads words from a dictionary file, and inserts them into a hash table. The dictionary file consists of a list of 62,454 correctly spelled lowercase words, separated by whitespace. The words are inserted into the hash table, with each bucket growing dynamically as necessary to hold all of the incoming data.. 1.Write a C program to accept 2 singly linked lists & print the elements which are common in both the lists. 2. Consider a linked list with n integers. Each node of the list is numbered from 1 to n. Develop a program using 'C' language to split this list into 4 lists so that: a. First list contains nodes numbered 1,5,9,13,_,_,_.
Hash Table Program in C - Tutorialspoint.
Cout<<" MENU: 1.Create"; cout<<" 2.Search for a value 3.Delete an value"; cout<<" Enter your choice:"; cin>>ch; switch(ch) {. case 1: cout<<" Enter the number of elements to be inserted:"; cin>>n. C program for dictionary using hashing,dictionary program in c language,c program to implement functions of. Implement the ADT dictionary by using hashing and separate chaining. Write a main program that demonstrates and tests this class. E Source Codes Software Programs C++ Programs Data structure C++ program to implement Hash.
GitHub - HimanshuSingal/dictionary_hashing: C++.
Use hcreate, hsearch and hdestroy to Implement Dictionary Functionality in C. Generally, the C standard library does not include a built-in dictionary data structure, but the POSIX standard specifies hash table management routines that can be utilized to implement dictionary functionality. Namely, hcreate, hsearch and hdestroy provide the. C Program To Implement Dictionary Using Hashing Meaning. C Program To Implement Dictionary Using Hashing Meaning Average ratng: 4,3/5 1838 votes. Hash table - Wikipedia. A small phone book as a hash table. In computing, a hash table (hash map) is a data structure used to implement an associative array, a structure that can map keys to values. A.
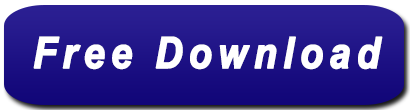
Dictionary using C - GitHub.
All of this gives us the ability to program some C++ code using an unordered map to implement a hash table, to give us these all in one guarantees as long as the load factor is correct. So now, you never have to write a hash table, you can just use the one in C++. I look forward to seeing some of your code as you work on using some hash tables. Jul 10, 2010 · I want to implement a dictionary using a hashtable. I have a "word" textfile that contains a list of over 100,000 words (each word is separated by a newline and the words are only composed of letters and apostrophes) that I'll have to load into memory, and another file that I'll have to spell check against the dictionary (this file can be composed of anything, so I'll only spell check the.
Hashing function in C | Types of Collision Resolution.
A hash table is a randomized data structure that supports the INSERT, DELETE, and FIND operations in expected O (1) time. The core idea behind hash tables is to use a hash function that maps a large keyspace to a smaller domain of array indices, and then use constant-time array operations to store and retrieve the data. 1. Dictionary data types. Writing a hashtable in C is a fun exercise -- every serious C programmer should do it at least once. – Lee Dec 8, 2010 at 5:24 I think of a dictionary being a datatype rather than a datastructure, since it could be implemented lots of ways -- a list, a hashtable, a tree, a self-balancing tree, etc. Are you asking for a dictionary, or a hashtable?.
Implementing a phone directory in C++ - Coding Ninjas Blog.
Ii) Write a C program for implementing Heap sort algorithm for sorting a given list of integers in ascending order. 35 10 Write a C program to implement all the functions of a dictionary (ADT) using hashing. 41 11 Write a C program for implementing Knuth -Morris - Pratt pattern matching algorithm. 46. Use Default Constructor to Create a Dictionary in C++. Alternatively, we can declare a map type object with given parameters and then initialize each key-value pair using a separate statement. In this sample, we demonstrate a map with int keys and the values of string -s. We can always put these statements in the loop or take values from the. Python dictionaries are implemented using hash tables. It is an array whose indexes are obtained using a hash function on the keys. The goal of a hash function is to distribute the keys evenly in the array. A good hash function minimizes the number of collisions e.g. different keys having the same hash.
Implement Dictionary in C - Delft Stack.
Launching Xcode. If nothing happens, download Xcode and try again. Launching Visual Studio Code. Your codespace will open once ready. There was a problem preparing your codespace, please try again. Latest commit. Jacob-C-Smith Simple dictionary implementation using MURMUR hash.. 44f4f97 25 minutes ago.
Algorithm and Examples of C++ Hash Table - EDUCBA.
C++ program to implement Stack using Formula Based Representation. Jul 10, 2010 implement dictionary with hashtable c++. I want to implement a dictionary using a hashtable. General C++ Programming; Lounge. Write a C Program to implement hashing.Hashing is the function or routine used to assign the key values to the each entity in the database. A HASH TABLE is a data structure that stores values using a pair of keys and values. Each value is assigned a unique key that is generated using a hash function. The name of the key is used to access its associated value. This makes searching for values in a hash table very fast, irrespective of the number of items in the hash table. 10 Write a C++ program to perform the following operations. a) Insertion into a AVL-tree b) Deletion from a AVL-tree 11 Write a C++ Program to implement all the functions of Dictionary (ADT) using hashing. 12 Write a C++ Program for implementing Knuth-Moris-Pratt pattern matching algorithm.
Hash Table in Data Structure: Python Example - Guru99.
Answer (1 of 4): Hello Everyone, Lets see how to implement a dictionary using C. The main goal of this code is to show malloc in use - dynamically allocating memory to dat structures. Hashing in the data structure is a technique of mapping a large chunk of data into small tables using a hashing function. It is also known as the message digest function. It is a technique that uniquely identifies a specific item from a collection of similar items. It uses hash tables to store the data in an array format.
Problem statement implement all the functions of a.
Lab 3 - Hash Table Spell Checking Due: Wednesday, February 8th th at 11:59PM Overview One straight-forward way to implement a fast spell checker is to use a hash table. If a word is found, it is assumed to be spelled correctly. Otherwise, it is assumed to be spelled incorrectly. Hash Table Program in C. Hash Table is a data structure which stores data in an associative manner. In hash table, the data is stored in an array format where each data value has its own unique index value. Access of data becomes very fast, if we know the index of the desired data. C Program To Implement Functions Of Dictionary Using Hashing You are to implement a simple hash table using C++. Your program will prompt for the name of an input file and the read and process the data contained in this file. The file contains a sequence of integer values. Read them and construct a hash table using chaining.
C Program To Implement Dictionary Using Hashing Algorithm.
C Program To Implement All The Functions Of A Dictionary (adt) Using Hashing GLib: by GNOME project. Several containers documented at: gnulib: by the GNU project. You are meant to copy paste the source. Problem Statement:-. Implement all the functions of a dictionary (ADT) using hashing. Data: Set of (key, value) pairs, Keys are mapped to values, Keys must be comparable, Keys must be unique Standard Operations: Insert (key, value), Find (key), Delete (key).
[Solved] C++ Hash Table In C++ , we will be implementing a.
Of data values. This technique is termed hashing, and the container built using this technique is called a hash table. The concept of hashing that we describe here is useful in implementing both Bag and Dictionary type collections. Much of our presentation will use the Bag as an example, as dealing with one value is easier than dealing with two. You can represent arbitrarily large numbers using arrays (constrained by available memory). But in order to perform calculations using arrays that represent numbers, you need to define the operations first. This is called "arbitrary precision arithmetic", and the C++ standard library doesn't provide an implementation of those operations..
See also:
International Fire Code 2015 Pdf Free Download
Sherlock Holmes Season 3 Download Torrent